Options Class Reference
#include <options.h>
Collaboration diagram for Options:
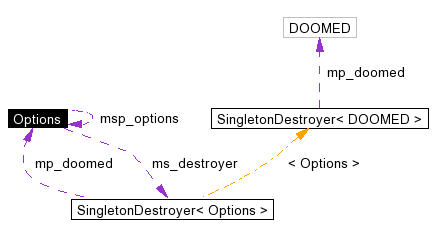
Public Member Functions | |
~Options () | |
Frees all the character strings. | |
int | filter () |
If true, Act as a pipe. | |
int | flush () |
If true, Flush on every write. | |
int | indent () |
If true, match user indentation. | |
int | noOp () |
If true, do nothing (not implemented). | |
int | printStates () |
If true, print state changes to output. | |
int | remove () |
If true, remove all traceStrings. | |
int | rtest () |
If true, regression testing. | |
int | verbose () |
If true, print file operations. | |
int | warnings () |
If true, print warnings (Duh!). | |
int | writeNames () |
If true, only write names to stderr. | |
const char * | patternFn () |
pattern file name | |
const char * | activeFn () |
active routine list file name | |
const char * | inactiveFn () |
inactive routine list file name | |
const char * | execDirName () |
exec dir name | |
const char * | defaultPatName () |
name of default pattern | |
const char * | tsMarker () |
Trace String default marker. | |
int | iarg () |
Current arg count. used to parse the file/dir arguements in main(). | |
const char * | operator[] (int i) |
index operator to get at GetPot argument list. | |
int | size () |
Total number of arguments. | |
void | printHelp (const char *application) |
Prints help and quits. | |
Static Public Member Functions | |
static Options & | instance () |
Returns the pointer as a Reference. build must be called first. | |
static void | build (int argc, char *argv[], const char *fn=".traceString") |
Used getpot.h to parse the argument list. | |
Private Member Functions | |
void | setPatternFn (const char *fn) |
save away the pattern file. | |
Options (int c, char *v[], const char *f) | |
Users can not construct one. | |
Options (const Options &) | |
Users can not copy one. | |
Options & | operator= (const Options &) |
Users can not assign one. | |
Private Attributes | |
unsigned int | m_iarg |
arg counter after options | |
int | m_rtest |
Rtest flag. | |
int | m_verbose |
Verbosity flag. | |
int | m_printStates |
Print change of state flag. | |
int | m_filter |
Use as a pipe flag. | |
int | m_writeNames |
Only write names flag. | |
int | m_noOp |
No op flag. | |
int | m_flush |
Flush after every call to fputs. | |
int | m_indent |
Match user indentation. | |
int | m_remove |
Remove all traceString code. | |
int | m_warnings |
Print Warnings. | |
char * | mp_patternFn |
Pattern file name. | |
char * | mp_execDirName |
Exec directory. | |
char * | mp_activeFn |
Active file name. | |
char * | mp_inactiveFn |
Inactive file name. | |
char * | mp_default |
Default trace String name. | |
char * | mp_tsMarker |
Default trace String marker. | |
GetPot * | mp_cl |
command line arguments | |
Static Private Attributes | |
static Options * | msp_options = 0 |
Singleton pointer. | |
static SingletonDestroyer< Options > | ms_destroyer |
Singleton destroyer. |
Detailed Description
- Description
- The idea for this class is to hold all the user options in one place. It is a singleton (with a singleton destroyer: SingletonDestroyer<DOOMED>). The public interface has just get functions.
The documentation for this class was generated from the following files:
- options.h
- options.C